GitHub Actions
How to integrate GitHub Actions with Cloudsmith
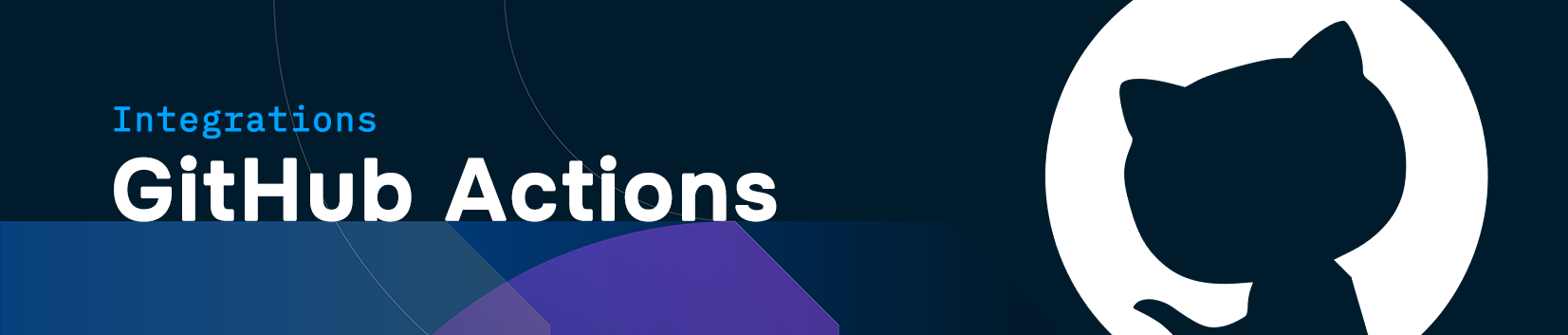
The Cloudsmith GitHub Action allows you to use the Cloudsmith CLI to upload/push packages to Cloudsmith repositories. It currently supports pushing:
- Alpine
- Cargo
- CocoaPods
- Composer
- Dart
- Debian
- Docker
- Go
- Helm
- Hex
- Maven
- npm
- NuGet
- Python
- RedHat/RPM
- Raw
Adding your API Key to GitHub
Retrieve your Cloudsmith API Key.
You will need to add a secret to your GitHub repository named CLOUDSMITH_API_KEY
, with the value of your API-Key. Secrets are added through your GitHub repository settings, please see the Creating and Storing Encrypted Secrets documentation on GitHub for further details.
Pass your CLOUDSMITH_API_KEY
secret to the Action as per the examples.
OIDC Authentication
When using OIDC with GitHub Actions, save the JWT token to an environment variable called
CLOUDSMITH_API_KEY
and do not includeapi-key
in the.yaml
push action - the API key will be taken from the environment variable instead.
Examples
Examples for all formats supported are available on the GitHub README.
Updated 11 months ago