Terraform Provider
How to integrate Terraform with Cloudsmith
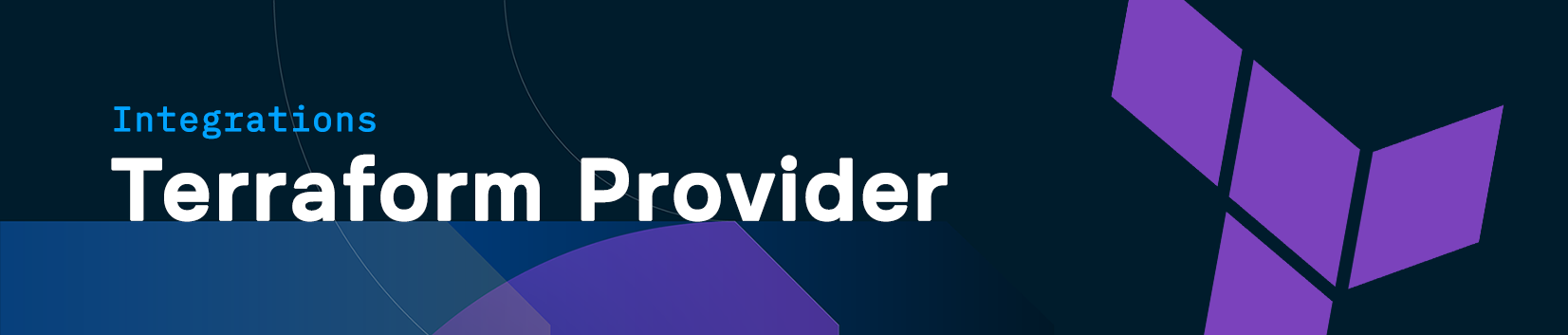
Cloudsmith is a verified provider on the Terraform Registry.
Installation
To install the Cloudsmith Terraform Provider, add the following to the required_providers
in your Terraform module:
required_providers {
cloudsmith = {
source = "cloudsmith-io/cloudsmith"
version = "0.0.60"
}
}
You also need to add your Cloudsmith API Key, for use by the provider:
provider "cloudsmith" {
api_key = "API-KEY"
}
You then run terraform init
and Terraform will automatically install the provider. To specify a particular provider version when installing providers, see the Terraform documentation on provider versioning.
Data Sources
namespace
The namespace data source allows fetching of metadata about a given Cloudsmith namespace. The fetched data can be used to resolve permanent identifiers from a namespace's user-facing name. These identifiers can then be passed to other resources to allow more consistent identification as user-facing names can change.
data "cloudsmith_namespace" "my_namespace" {
slug = "my-namespace"
}
Argument | Description | Required |
---|---|---|
slug | The slug identifies the namespace in URIs | Yes |
Resources
repository
The repository resource allows the creation and management of package repositories within a Cloudsmith namespace. Repositories store packages and are the main entities with which Cloudsmith users interact.
resource "cloudsmith_repository" "my_repository" {
name = "My Repository"
namespace = "${data.cloudsmith_namespace.my_namespace.slug_perm}"
description = "A certifiably-awesome private package repository"
}
Argument | Description | Required |
---|---|---|
name | A descriptive name for the repository. | Yes |
namespace | Namespace / account to which this repository belongs. | Yes |
description | A description of the repository's purpose/contents. | No |
index_files | If checked, files contained in packages will be indexed, which increase the synchronisation time required for packages. Note that it is recommended you keep this enabled unless the synchronisation time is significantly impacted. | No |
repository_type | Private repositories are visible only to users that have been granted access. Public repositories are visible to all Cloudsmith users. | No |
slug | The slug identifies the repository in URIs. | No |
storage_region | The Cloudsmith region in which package files are stored. Valid values are:default, ie-dublin, de-frankfurt, ca-montreal, us-norcal, us-ohio, us-oregon, sg-singapore, au-sydney | No |
wait_for_deletion | If true, terraform will wait for a repository to be permanently deleted before finishing. | No |
See the repository documentation for more information on creating and managing repositories.
repository privileges
The repository privileges resource allows the management of privileges for a given Cloudsmith repository. Using this resource it is possible to assign users, teams, or service accounts to a repository, and define the appropriate permission level for each.
Note that while users can be added to repositories in this manner, since Terraform does not (and cannot currently) manage those user accounts, you may encounter issues if the users change or are deleted outside of Terraform.
resource "cloudsmith_repository_privileges" "privs" {
organization = data.cloudsmith_organization.my_organization.slug
repository = cloudsmith_repository.my_repository.slug
service {
privilege = "Write"
slug = cloudsmith_service.my_service.slug
}
team {
privilege = "Write"
slug = cloudsmith_team.my_team.slug
}
team {
privilege = "Read"
slug = cloudsmith_team.my_other_team.slug
}
user {
privilege = "Read"
slug = "some-user-slug"
}
}
When creating a repository via Terraform using a service account and subsequently adjusting the privileges on that same repository, ensure to include a block giving the provisioning account the 'Admin' privilege (as it would get by default by being the creator). This will ensure that the account can continue to provision resources (e.g. entitlements). Otherwise, the resource may overwrite that privilege and further provisioning on the repository when using that account would fail.
Argument | Description | Required |
---|---|---|
organization | Organization to which this repository belongs. | Yes |
repository | Repository to which these privileges apply. | Yes |
service | Namespace / Account to which this token belongs | No |
privilege | The service's privilege level in the repository. Must be one of Admin, Write, or Read. | Yes |
slug | The slug/identifier of the service. | Yes |
team | Variable number of blocks containing teams that should have repository privileges. | No |
privilege | The team's privilege level in the repository. Must be one of Admin, Write, or Read. | Yes |
slug | The slug/identifier of the team. | Yes |
user | Variable number of blocks containing users that should have repository privileges. | No |
privilege | The user's privilege level in the repository. Must be one of Admin, Write, or Read. | Yes |
slug | The slug/identifier of the user. | Yes |
entitlement
The entitlement resource allows the creation and management of Entitlement tokens for a Cloudsmith repository. Entitlement tokens grant read-only access to a repository and can be configured with a number of custom restrictions if necessary.
resource "cloudsmith_entitlement" "my_entitlement" {
name = "Entitlement Token 1"
namespace = "${cloudsmith_repository.test.namespace}"
repository = "${cloudsmith_repository.test.slug_perm}"
}
Argument | Description | Required |
---|---|---|
name | A descriptive name for the token | Yes |
namespace | Namespace / Account to which this token belongs | Yes |
repository | Repository to which this token belongs | Yes |
token | The literal string value of the token to be created | No |
is_active | If true, the token will be enabled and will allow downloads based on configured restrictions (if any). | No |
limit_date_range_from | The starting date/time the token is allowed to be used from. | No |
limit_date_range_to | The ending date/time the token is allowed to be used to. | No |
limit_num_clients | The maximum number of unique clients allowed for the token. | No |
limit_num_downloads | The maximum number of downloads allowed for the token. | No |
limit_package_query | The package-based search query to apply to restrict downloads to. This uses the same syntax as the standard search used for repositories, and also supports boolean logic operators such as OR/AND/NOT and parentheses for grouping. This will still allow access to non-package files, such as metadata. | No |
limit_path_query | The path-based search query to apply to restrict downloads to. This supports boolean logic operators such as OR/AND/NOT and parentheses for grouping. The path evaluated does not include the domain name, the namespace, the entitlement code used, the package format, etc. and it always starts with a forward slash. | No |
Please see the Entitlements documentation for more details on managing entitlement tokens and restrictions.
Example Module
A complete, but minimal, example of a Terraform module that uses the Cloudsmith Provider to create a repository and an Entitlement Token (with some basic token restrictions) is:
terraform {
required_providers {
cloudsmith = {
source = "cloudsmith-io/cloudsmith"
version = "0.0.5"
}
}
}
provider "cloudsmith" {
api_key = "abcdefghijlkl1234567890"
}
data "cloudsmith_namespace" "demo-organization {
slug = "demo-org"
}
resource "cloudsmith_repository" "terraform-demo" {
description = "Example repo provisioned by Terraform"
name = "Terraform Demo"
namespace = "${data.cloudsmith_namespace.demo.slug_perm}"
slug = "terraform-demo"
repository_type = "Private"
}
resource "cloudsmith_entitlement" "demo-entitlement" {
name = "Token 1"
namespace = "${cloudsmith_repository.terraform-demo.namespace}"
repository = "${cloudsmith_repository.terraform-demo.slug_perm}"
limit_num_downloads = "15"
limit_num_clients ="1"
limit_package_query = "name:,my-package"
}
More example modules
Further examples can be found on github in our terraform-provider: https://github.com/cloudsmith-io/terraform-provider-cloudsmith/tree/master/examples
Updated about 2 months ago